Polars, DuckDB, Pandas, Modin, Ponder, Fugue, Daft — which one is the best dataframe and SQL tool?
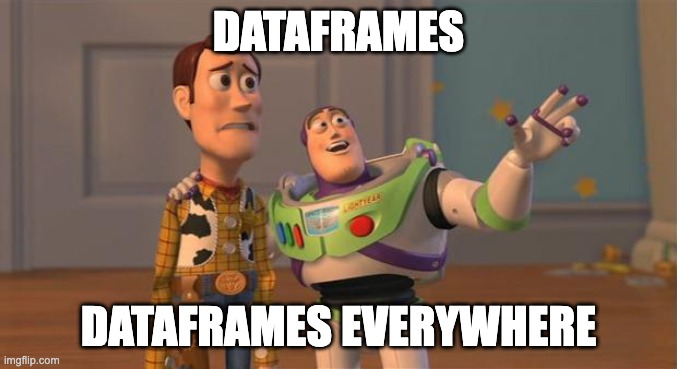
Comparing open-source dataframe and SQL frameworks for data engineering, machine learning and analytics
Comparing open-source dataframe and SQL frameworks for data engineering, machine learning and analytics
Stay up to date with the latest features and changes to Kestra