Generating Fake Data to Create End-to End Orchestration Projects
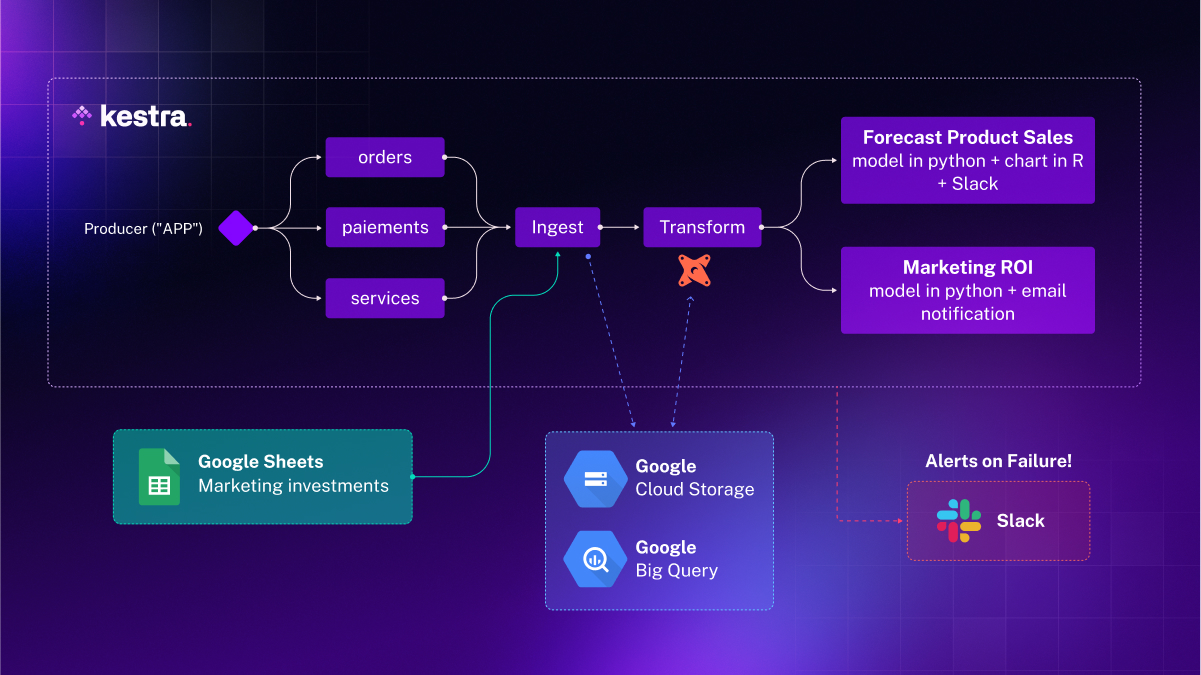
Generates dynamic fake data for end-to-end orchestration projects with Kestra, for realistic data use cases and hands-on experience
Generates dynamic fake data for end-to-end orchestration projects with Kestra, for realistic data use cases and hands-on experience
Stay up to date with the latest features and changes to Kestra